By removing assignments to parameters within a function or method, the “Remove Assignments to Parameters” refactoring approach helps to make code more readable and maintainable. By substituting local variables for the assignments, the code is made more transparent and less prone to errors.
Ways to Apply Remove Assignments to Parameters Technique
Here’s a step-by-step guide on how to apply the “Remove Assignments to Parameters” refactoring technique:
- Determine the procedure or function where parameter assignments take place. Search the function body for any parameter that is receiving a new value.
- Within the function or method, declare a new local variable and give it the parameter’s value. Give the local variable a name that accurately reflects its function.
- In the body of the code, replace every instance of the parameter with the newly formed local variable
- Update all function calls that send arguments to the refactored function and any references to the parameter in the function signature. These ought should now substitute the new local variable for the old argument.
- Repeat the process for each parameter being assigned within the function or method.
- Check the code to ensure the function’s or method’s behavior hasn’t changed. Make that the local variables are being used correctly and that any changes you make don’t have any unwanted consequences.
- To make sure the refactored code still performs as planned, extensively test it.
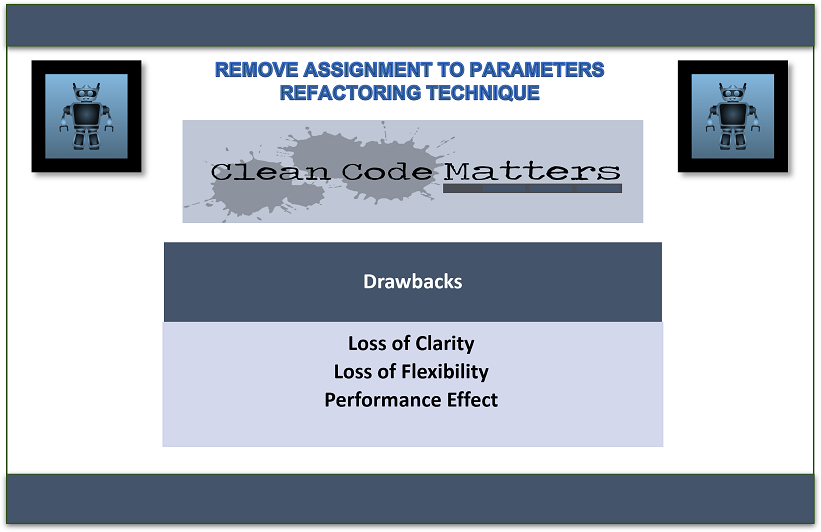
Problem
Inside the method’s body, a parameter is given or given a value.
Before Refactoring
def calculate_discount(price, discount_rate):
if price > 100:
discount_rate = 0.2 # Assigning a new value to the discount_rate parameter
else:
discount_rate = 0.1 # Assigning a different value to the discount_rate parameter
discounted_price = price - (price * discount_rate)
return discounted_price
Solution
Use a local variable in place of a parameter.
After Refactoring
def calculate_discount(price, discount_rate):
if price > 100:
local_discount_rate = 0.2 # Using a local variable instead of assigning to the parameter
else:
local_discount_rate = 0.1
discounted_price = price - (price * local_discount_rate)
return discounted_price
In the “before” code, the discount_rate
the parameter is being reassigned within the function, which can lead to confusion and potential bugs. The refactored “after” code creates a local variable local_discount_rate
and assigns it the appropriate value based on the condition. The local variable is then used in the calculation, keeping the original parameter intact.
The code is made easier to read and less likely to contain errors by deleting the assignments to the parameter. It makes the code more maintainable by separating the input parameters from the local variables used within the method.
Drawbacks of Removing Assignments to Parameters Refactoring Technique
While using the “Remove Assignments to Parameters” refactoring technique might make code easier to understand and maintain, it’s vital to think about any potential negatives and how to work around them. The following are some downsides and solutions for them:
- Increased memory usage: When local variables are used in place of parameter assignments, the amount of memory used may rise. Memory space is taken up by each local variable. However, unless you’re working with a lot of function calls or very little memory, this increase in memory use is typically insignificant.
- Code redundancy is a possibility in some situations where local variables are used to replace parameter assignments. There may be numerous local variables with the same value if the parameter is used more than once in the function. Instead of creating a local variable to fix this, you might think about utilizing the original parameter directly where it is required.
- Consistency: It’s crucial to maintain consistency across the entire codebase when using the refactoring technique on a function. To maintain consistency, you should consider whether to restructure the other functions or methods that use the same parameter or leave the assignments in place. Applying the refactoring technique erratically can make it more difficult to comprehend and manage the codebase.
Resolving Strategy
Here are some problem-solving techniques to handle the potential downsides of the “Remove Assignments to Parameters” refactoring technique:
- Memory usage: By using the refactoring technique judiciously, you can reduce the increased memory usage if it becomes an issue. Concentrate on the functions or methods where the advantages of eliminating parameter assignments outweigh the memory impact. As long as they don’t cause complications or problems, assignments to parameters can be kept in less important or performance-sensitive code.
- Redundancy: Examine how the argument is used within the function to prevent the creation of redundant local variables. It can be preferable to preserve the assignments rather than adding a new local variable if the parameter is only used once or in a few select locations. However, utilizing a local variable can still enhance readability and maintainability if the parameter is used frequently.
- Consistency: The maintainability of a codebase depends on consistency. If you choose to restructure one function, search the code base for other functions that are comparable to it and use the same parameter. Consider implementing the refactoring technique uniformly across the codebase or, for consistency, explain and express the choice to retain assignments in some circumstances.
You can also visit other blogs to better understand the most recent hot topics in technology.