In computer science or programming, the phrase “split temporary variable” does not have a defined or well-known meaning. It might be a phrase used within a specific programming language or framework that I am not familiar with, or it might have a specific context.
However, judging by the words themselves, it might also mean dividing a temporary variable into a number of components or segments. To accomplish this, it may be necessary to divide the value kept in a temporary variable and assign various parts of it to various variables or data structures.
It is difficult to give a more detailed explanation or comprehension of what the word “split temporary variable” means in the absence of more context or clarity. I’d be pleased to try and help you further if you could provide me additional details regarding the context or application of this term.
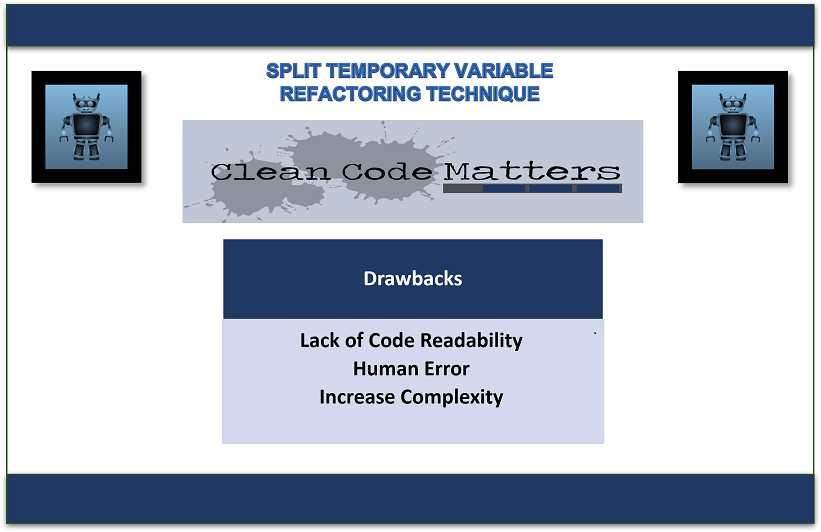
Ways to Apply Split Temporary Variable Technique
A step-by-step tutorial for using the “Split Temporary Variable” refactoring approach is provided below:
- Find the temporary variable by looking for a variable that is utilized multiple times within the same block or procedure of code.
- Analyze the various aims: Identify the many functions or duties performed by the temporary variable in the code. Is the same variable being used for many activities or calculations?
- Create distinct variables: Make new variables with evocative names for each unique function that the temporary variable serves.
- Assign values: Use the newly generated variables you produced in the preceding step in lieu of the original temporary variable’s usages. Depending on the purpose of each variable, give it the proper values.
- Check to see if the code still works as intended following the modifications. Run tests to make sure the refactoring hasn’t changed the behavior.
Problem
You have a local variable in your code that stores values many times.
Before Refactoring
def calculate_total_amount(quantity, price):
total = quantity * price
discount = 0.1 * total
total = total - discount
# Some other calculations or operations using the 'total' variable
return total
In this code, the total
variable is used both to calculate the total amount and to store the discounted amount. To apply the “Split Temporary Variable” refactoring, we can separate these two purposes into distinct variables.
Solution
Multiple separate variables are used in place of a single variable to store results.
After Refactoring
def calculate_total_amount(quantity, price):
total_amount = quantity * price
discount = 0.1 * total_amount
discounted_total = total_amount - discount
# Some other calculations or operations using the 'discounted_total' variable
return discounted_total
In the refactored code, we have split the original total
variable into two separate variables: total_amount
and discounted_total
. Now, the total_amount
variable represents the initial calculation of the total amount, while the discounted_total
variable holds the final amount after applying the discount.
The temporary variable is divided, making the code easier to read and understand. Since each variable serves a distinct role, it is simpler to comprehend the code’s intended meaning. Additionally, each particular value can be separately modified or subject to additional calculations without impacting the others.
Drawbacks of Split Temporary Variable Refactoring Technique
Although the “Split Temporary Variable” refactoring technique can often increase the readability and maintainability of code, it’s crucial to be aware of any potential risks or issues. Here are a few potential negatives:
- Memory consumption may increase as a result of dividing a temporary variable into numerous variables. Splitting a temporary variable into many variables could significantly raise the total memory footprint of the code, even if the original temporary variable was only sometimes utilized and had a small scope.
- Code duplication: If the same value needs to be utilized in many locations after splitting a temporary variable, this could result in code repetition. In certain circumstances, updating several variables consistently may be necessary, which, if improperly managed, could lead to inconsistencies or errors
- Maintenance burden: Dividing a temporary variable can result in more variables being used throughout the code. This might necessitate more work in terms of variable naming, documentation, and comprehension of roles. Additionally, it can make upcoming alterations or refactorings more difficult and error-prone.
- Splitting a temporary variable may have an effect on the execution time or computational efficiency in some performance-sensitive cases. Overhead could be introduced by adding more variables and performing more assignments. Before assuming a large performance disadvantage, it’s crucial to quantify and evaluate the real impact in the particular circumstance.
Resolving Strategy
You can think about the following solutions to deal with the potential downsides of the “Split Temporary Variable” refactoring technique:
- Memory optimization: If memory utilization is an issue, you might assess if the extra memory usage caused by splitting the temporary variable is noticeable or insignificant in your particular situation. If it turns out to be a problem, you might look at other options like reusing variables whenever you can or maximizing memory use in other areas of the code.
- Code abstraction: When separating a temporary variable, think about abstracting common actions or calculations into reusable functions or methods to prevent code duplication. This can lessen duplication and guarantee regular updates for various variables.
- Documentation and naming conventions: It is essential to give each variable a distinct, meaningful name as the number of variables grows after splitting. To make it easier to comprehend and maintain each variable, list its duties and purposes. Additionally, consistent naming practices might aid in making code easier to read.
- Performance analysis: If performance is an issue, carefully consider how dividing the temporary variable will affect things in your particular situation. To ascertain whether the added overhead is significant or insignificant, measure and profile the code. If necessary, take into account alternate strategies or improvements, such as reorganizing the code or making algorithmic changes.
In the end, overcoming any refactoring technique’s possible downsides requires rigorous study, evaluation of trade-offs, and alignment of the choice with the particular requirements and restrictions of your project.
You may read our other blogs here: