A section of code from a larger method is moved to a new, smaller method using an extract method. Because the new method can be called from the original method, the code will then be easier to understand and maintain.
How to Apply the Extract Method Refactoring Technique?
Applying the Extract Method refactoring technique involves the following steps:
- Find a section of the code that carries out a clear, unified function. This could be a section of code that runs a computation, a loop that iterates through a set of objects, or a series of data manipulation actions.
- Pick a name for the new method that accurately describes what it does. The name should appropriately describe the function of the extracted code and be both short and clear.
- The extracted code should be moved into the newly created method in the class where the original code was placed. Any required parameters should be passed to the new method, and any necessary values should be returned to the original method.
- Call the new method by modifying the previous one, supplying any required arguments, and utilizing the return value as necessary.
- Make that the updated code still functions properly by testing it.
- Refactor the new method as appropriate to make it more readable, maintainable, and reusable. This can entail changing variables, enhancing the code’s structure, or adding comments to make it clearer.
- To divide other cohesive code sections into different methods, repeat the process as necessary.
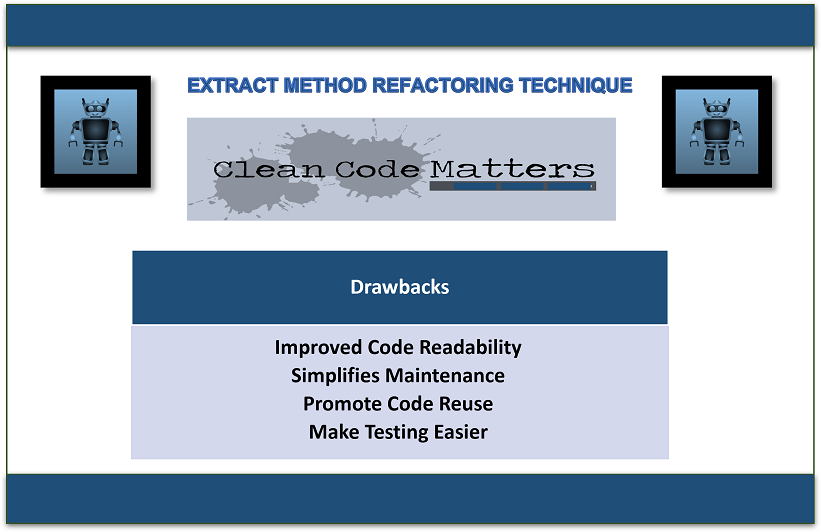
Problem
You have a collection of separate pieces of code.
Before Refactoring
def calculate_total_price(products):
total_price = 0
for product in products:
price = product.price
quantity = product.quantity
subtotal = price * quantity
total_price += subtotal
return total_price
Solution
Put this code in a distinct new method (or function) and call the new method instead of the previous code.
After Refactoring
def calculate_total_price(products):
total_price = 0
for product in products:
subtotal = calculate_subtotal(product)
total_price += subtotal
return total_price
def calculate_subtotal(product):
price = product.price
quantity = product.quantity
subtotal = price * quantity
return subtotal
In the refactored code, the logic to calculate the subtotal of a single product has been extracted into a separate method called calculate_subtotal()
. The calculate_total_price()
method now calls this new method for each product in the list, making the code easier to read and understand. Additionally, if the logic for calculating the subtotal needs to be changed in the future, it can be modified in a single place within the calculate_subtotal()
method, rather than having to change it multiple times within the calculate_total_price()
method.
Drawbacks of the Extract Method Refactoring Technique
There are several disadvantages to the Extract Method refactoring approach that you should be aware of even if it can be useful for enhancing the readability, maintainability, and reusability of code. Here are a few possible negatives:
- Increased complexity: The complexity of the codebase as a whole may grow if you extract too many methods from a single larger method. Debugging problems and comprehending the program’s logic may become more challenging as a result.
- Poor method design: If the new method you are extracting is not well designed, it may not be as valuable or reusable as you had intended. It’s crucial to give the method appropriate names, choose the input and output parameters with care, and make sure the method completes a single, coherent purpose.
- Increased number of method calls: You can increase the number of methods calls in your code by extracting it into numerous methods. Performance may be slightly impacted by this, particularly if the extracted method is regularly invoked.
Resolving Strategy
Here are some tactics you can use to lessen these drawbacks:
- Keep the extracted methods concise: A new method should be extracted as succinctly and narrowly as feasible. This will assist in reducing the codebase’s overall complexity.
- Utilize good method design concepts: To make the new method simple to comprehend and reusable, utilize good software engineering principles like the Single Responsibility Principle (SRP).
- Balance the number of method calls: If you see that the number of method calls has greatly increased, you can attempt to make up for this by utilizing other refactoring approaches, such as Inline Method or Collapse Hierarchy.
- Write automated tests: It’s crucial to write automated tests when you restructure your code to make sure the functionality is preserved. This can aid in identifying problems brought on by a method’s poor design or rising complexity.
You can also visit other blogs to better understand the most recent hot topics in technology.
One Comment on “Extract Method | Drawbacks and Resolving Strategy”